Compare Two Strings in C++
To compare two string in C++ Programming, you have to ask to the user to enter the two string and start comparing using the function strcmp(). If it will return 0, then both string will be equal and if it will not return 0, then both string will not be equal to each other as shown in here in the following program.
C++ Programming Code to Compare Two String
Following C++ program ask the user to enter two string to check whether the two strings are equal or not using the strcmp() function of string.h library.
Here, strcmp() function takes two argument like strcmp(str1, str2) to compare the two string to check whether it is equal or not. If the two strings are equal then it will return 0, otherwise if the two strings are not equal then it will return 1. So 0 for equal and 1 for not equal.
/* C++ Program - Compare Two String */ #include<iostream.h> #include<conio.h> #include<string.h> #include<stdio.h> void main() { clrscr(); char str1[100], str2[100]; cout<<"Enter first string : "; gets(str1); cout<<"Enter second string : "; gets(str2); if(strcmp(str1, str2)==0) { cout<<"Both the strings are equal"; } else { cout<<"Both the strings are not equal"; } getch(); }
When the above C++ program is compile and executed, it will produce the following result. Above C++ Programming Example Output (for equal strings):
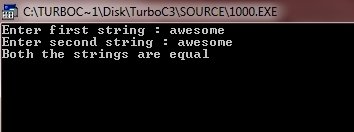
Above C++ Programming Example Output (for unequal strings):
