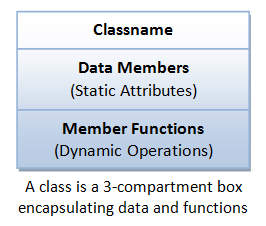
A class can be visualized as a three-compartment box, as illustrated:
In other words, a class encapsulates the static attributes (data) and dynamic behaviors (operations that operate on the data) in a box.
Class Members: The data members and member functions are collectively called class members.
The followings figure shows a few examples of classes:
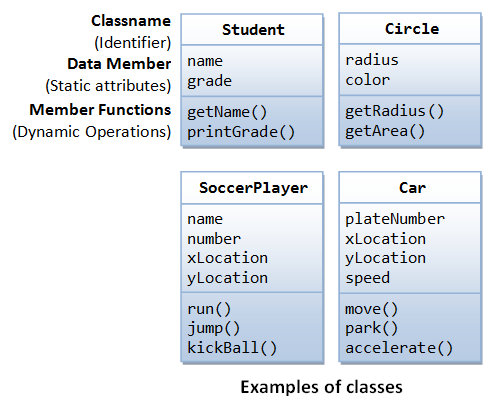
The following figure shows two instances of the class
Student
, identified as "paul
" and "peter
".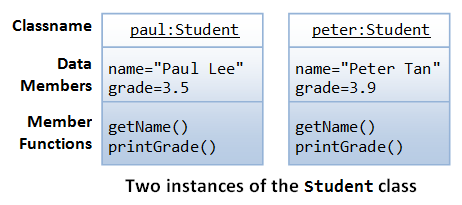
Unified Modeling Language (UML) Class and Instance Diagrams: The above class diagrams are drawn according to the UML notations. A class is represented as a 3-compartment box, containing name, data members (variables), and member functions, respectively. classname is shown in bold and centralized. An instance (object) is also represented as a 3-compartment box, with instance name shown as
instanceName:Classname
and underlined.Brief Summary
- A class is a programmer-defined, abstract, self-contained, reusable software entity that mimics a real-world thing.
- A class is a 3-compartment box containing the name, data members (variables) and the member functions.
- A class encapsulates the data structures (in data members) and algorithms (member functions). The values of the data members constitute its state. The member functions constitute its behaviors.
- An instance is an instantiation (or realization) of a particular item of a class.
2.4 Creating Instances of a Class
To create an instance of a class, you have to:
- Declare an instance identifier (name) of a particular class.
- Invoke a constructor to construct the instance (i.e., allocate storage for the instance and initialize the variables).
For examples, suppose that we have a class called
Circle
, we can create instances of Circle
as follows:// Construct 3 instances of the class Circle: c1, c2, and c3 Circle c1(1.2, "red"); // radius, color Circle c2(3.4); // radius, default color Circle c3; // default radius and color
Alternatively, you can invoke the constructor explicitly using the following syntax:
Circle c1 = Circle(1.2, "red"); // radius, color Circle c2 = Circle(3.4); // radius, default color Circle c3 = Circle(); // default radius and color
2.5 Dot (.) Operator
To reference a member of a object (data member or member function), you must:
- First identify the instance you are interested in, and then
- Use the dot operator (
.
) to reference the member, in the form ofinstanceName.memberName
.
For example, suppose that we have a class called
Circle
, with two data members (radius
and color
) and two functions (getRadius()
and getArea()
). We have created three instances of the class Circle
, namely, c1
, c2
and c3
. To invoke the function getArea()
, you must first identity the instance of interest, says c2
, then use the dot operator, in the form of c2.getArea()
, to invoke the getArea()
function of instance c2
.
For example,
// Declare and construct instances c1 and c2 of the class Circle Circle c1(1.2, "blue"); Circle c2(3.4, "green"); // Invoke member function via dot operator cout << c1.getArea() << endl; cout << c2.getArea() << endl; // Reference data members via dot operator c1.radius = 5.5; c2.radius = 6.6;
Calling
getArea()
without identifying the instance is meaningless, as the radius is unknown (there could be many instances of Circle
- each maintaining its own radius).
In general, suppose there is a class called
AClass
with a data member called aData
and a member function called aFunction()
. An instance called anInstance
is constructed for AClass
. You use anInstance.aData
and anInstance.aFunction()
.2.6 Data Members (Variables)
A data member (variable) has a name (or identifier) and a type; and holds a value of that particular type (as descried in the earlier chapter). A data member can also be an instance of a certain class (to be discussed later).
Data Member Naming Convention: A data member name shall be a noun or a noun phrase made up of several words. The first word is in lowercase and the rest of the words are initial-capitalized (camel-case), e.g.,
fontSize
, roomNumber
, xMax
, yMin
and xTopLeft
. Take note that variable name begins with an lowercase, while classname begins with an uppercase.2.7 Member Functions
A member function (as described in the earlier chapter):
- receives parameters from the caller,
- performs the operations defined in the function body, and
- returns a piece of result (or void) to the caller.
Member Function Naming Convention: A function name shall be a verb, or a verb phrase made up of several words. The first word is in lowercase and the rest of the words are initial-capitalized (camel-case). For example,
getRadius()
, getParameterValues()
.
Take note that data member name is a noun (denoting a static attribute), while function name is a verb (denoting an action). They have the same naming convention. Nevertheless, you can easily distinguish them from the context. Functions take arguments in parentheses (possibly zero argument with empty parentheses), but variables do not. In this writing, functions are denoted with a pair of parentheses, e.g.,
println()
, getArea()
for clarity.2.8 Putting them Together: An OOP Example
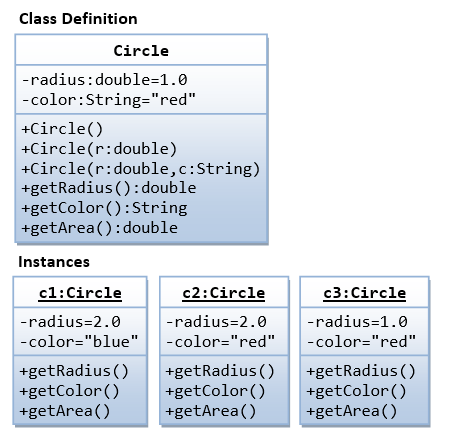
A class called
Circle
is to be defined as illustrated in the class diagram. It contains two data members: radius
(of type double
) and color
(of type String
); and three member functions: getRadius()
, getColor()
, and getArea()
.
Three instances of
Circle
s called c1
, c2
, and c3
shall then be constructed with their respective data members, as shown in the instance diagrams.
In this example, we shall keep all the codes in a single source file called
CircleAIO.cpp
.CircleAIO.cpp
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 | /* The Circle class (All source codes in one file) (CircleAIO.cpp) */ #include <iostream> // using IO functions #include <string> // using string using namespace std; class Circle { private: double radius; // Data member (Variable) string color; // Data member (Variable) public: // Constructor with default values for data members Circle(double r = 1.0, string c = "red") { radius = r; color = c; } double getRadius() { // Member function (Getter) return radius; } string getColor() { // Member function (Getter) return color; } double getArea() { // Member function return radius*radius*3.1416; } }; // need to end the class declaration with a semi-colon // Test driver function int main() { // Construct a Circle instance Circle c1(1.2, "blue"); cout << "Radius=" << c1.getRadius() << " Area=" << c1.getArea() << " Color=" << c1.getColor() << endl; // Construct another Circle instance Circle c2(3.4); // default color cout << "Radius=" << c2.getRadius() << " Area=" << c2.getArea() << " Color=" << c2.getColor() << endl; // Construct a Circle instance using default no-arg constructor Circle c3; // default radius and color cout << "Radius=" << c3.getRadius() << " Area=" << c3.getArea() << " Color=" << c3.getColor() << endl; return 0; } |
To compile and run the program (with GNU GCC under Windows):
> g++ -o CircleAIO.exe CircleAIO.cpp
// -o specifies the output file name
> CircleAIO
Radius=1.2 Area=4.5239 Color=blue
Radius=3.4 Area=36.3169 Color=red
Radius=1 Area=3.1416 Color=red